About a year ago, Sophie Alpert and Dan Abramov presented one of the most exciting new features in React: Hooks. Release 16.8 introduced several alternatives that can be used directly (e.g. useState
, useEffect
and some others), but also provided the possibility to create your very own hooks – Custom Hooks.
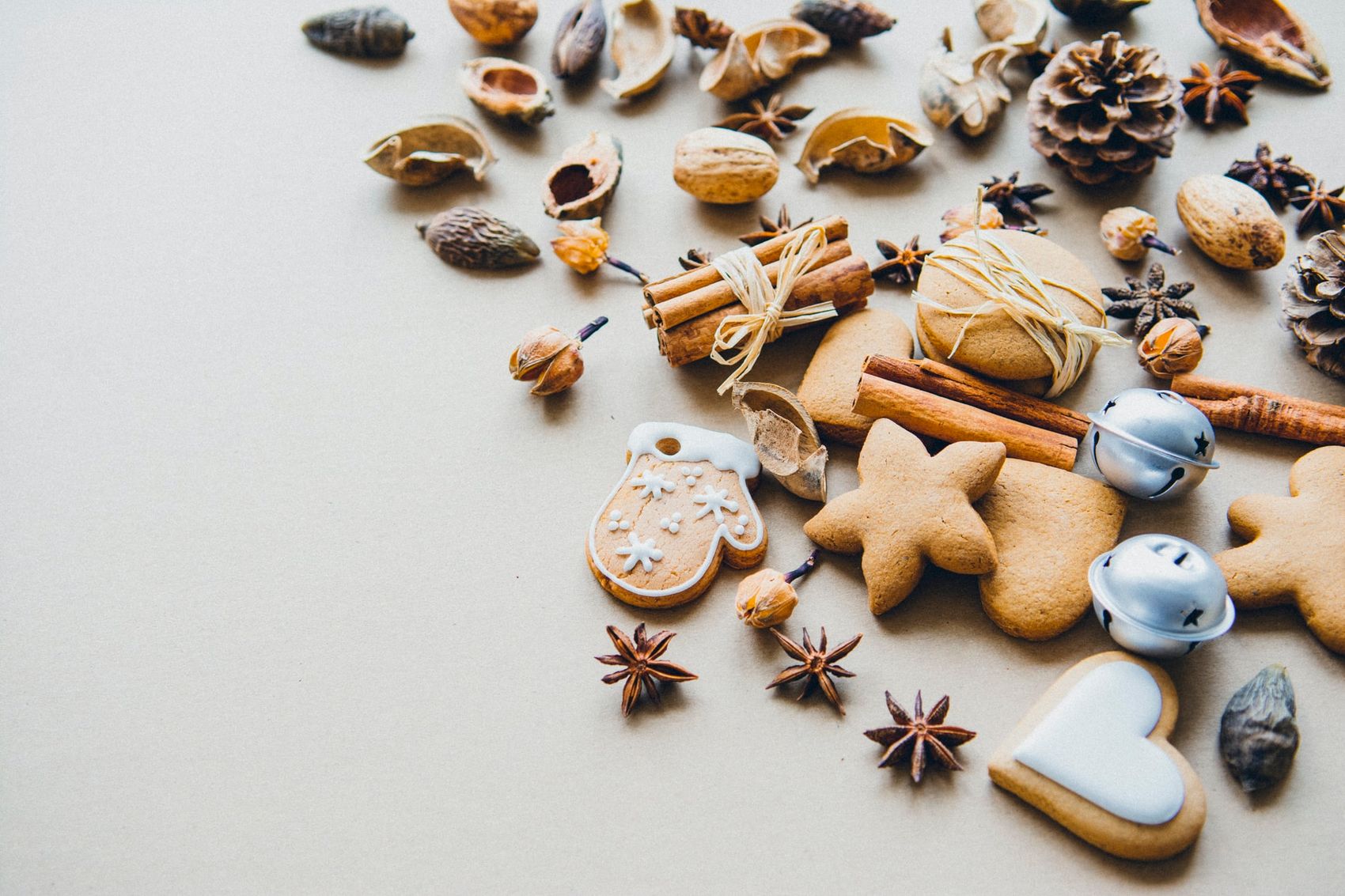
Custom Hooks anatomy
Suppose you want to create an app that keeps track of a value even after a page refresh. This can easily be done by taking advantage of the browser's localStorage. A Custom Hook for this may look like the following:
import React, { useState, useEffect } from 'react';
function useLocalStorage = (key, initialValue) => {
const [value, setValue] = useState(
() => window.localStorage.getItem(key) || initialValue
);
useEffect(() => {
window.localStorage.setItem(key, value);
}, [key, value]);
return { value, setValue };
};
Like all the pre-defined hooks in the react
package, a Custom Hook's name should always start with the phrase "use", making them distinguishable from other concepts in our application. Custom Hook sounds tough, but luckily for us it's really just a fancy way of saying "a function containing other hooks and/or some additional logic" (and remember: Hooks don't work in class components).
If you would like to use the useLocalStorage
hook from above, you could easily do so like this:
const dayFromLocalStorage = useLocalStorage("day", 13);
dayFromLocalStorage.value; // day: 13
dayFromLocalStorage.setValue(24); // day: 24
The whole idea behind a Custom Hook is to support the reuse of stateful logic and to abstract the implementation of something frequently used in your application (acts as a black box).
Popular Custom Hooks
At our client project, we use several Custom Hooks in our daily work. The most important aspect of using them is that they solve a typical problem in the application. These abstractions make sense for us in our daily work. However, there are some patterns that may be recognised even across projects, domains and countries. This is where popular Custom Hook packages come into play. Fortunately for us, many have already started to develop comprehensive abstractions and black boxes for us. Let's take a look at the most popular:
useForm – Ever struggled with state in form validations? It can really help you manage the state of your forms.
react-use – Not a single hook, but a collection of many different (contains hooks for interacting with sensors, UI, state etc.)
useHooks.com – This website presents third-party Hooks every once in a while with simple but detailed examples.
Still, it is a joyful task to create your own Custom Hooks. For example, have a look at how you can make setInterval declarative with Hooks, or check out what people already have done in other awesome react hook packages.
And remember, by creating your very own Custom Hooks, you will automatically be just as cool as the redux
and react-router-dom
npm packages, who recently added several hooks. Ho Ho Hoooooooks! 👊🎄