Most React apps use JSX - but how can you set up a JSX environment yourself?
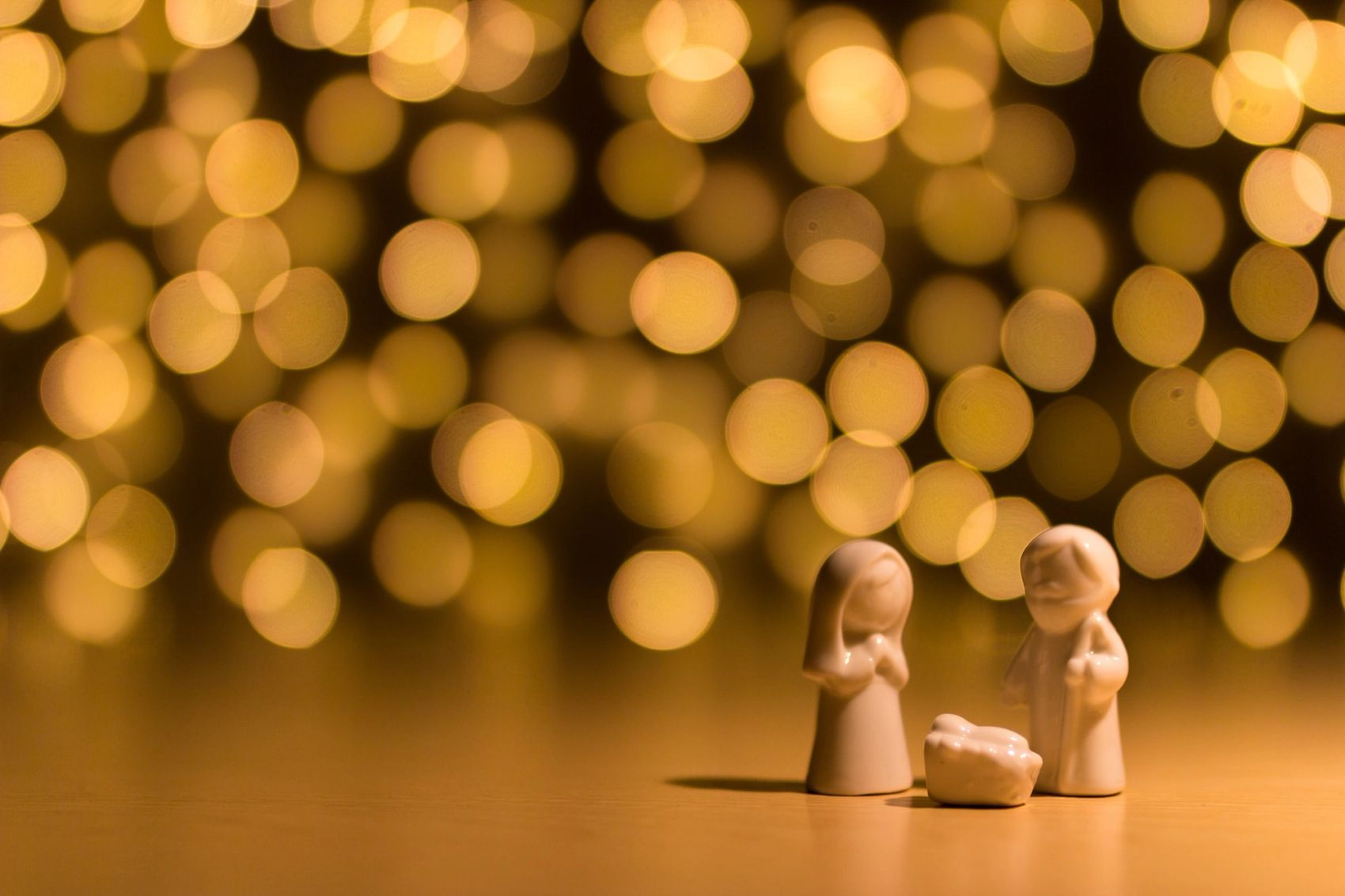
JSX is a syntax extension to JavaScript, that's a funny blend between JavaScript and a templating language. It's what most developers use to create React apps, and if you're on day 23 of this React calendar, I'm pretty sure you've at least seen it before.
JSX isn't a part of JavaScript, though, so how can we make it compile in our browsers? This article will show you five ways to get there.
1: Fuck JSX ๐
The first way to use JSX is to... not use it at all. You don't need JSX, and JSX doesn't need you. All JSX is, is syntactical sugar for React.createElement
! In other words, this code snippet:
const Santa = <div className="big-santa">๐
</div>;
is compiled down to this:
const Santa = React.createElement("div", { className: "big-santa" }, "๐
");
(don't believe me? Check out and play with the REPL!)
You can write and ship this right away - no compilation needed. You can read more about this approach in the documentation!
2: Do it live! ๐ข
If you're in a hurry, doing a workshop or for whatever reason don't have time to deal with setting up a build step for your app, there's actually a runtime build of Babel you can use if you want. You can add it by adding this line to your HTML:
<script src="https://unpkg.com/babel-standalone@6/babel.min.js" />
Next, you'll have to write your script tags like this:
<script type="text/babel">
// JSX is parsed here
const Hello = () => <div>Hello world</div>;
</script>
You can read more about this approach in the documentation too! (Pro-tip: Even if you're an experienced React developer, read the docs from top to bottom. They're really great!)
3: Use webpack! ๐พ
When you're done experimenting, you typically want something a bit more efficient than runtime compilation. That's why we end up using build tools like webpack
.
webpack
lets you use babel
- a JavaScript transpilation tool - to turn your JSX into runnable JavaScript again. You can add it by adding the following to your webpack.config.js
file:
{
module: {
rules: [{ test: /.js$/, use: "babel-loader" }];
}
}
Then you need to add a file called babel.config.js
with the following as well:
export default {
presets: ["@babel/react"],
};
There are great tutorials out there that details this process, if you're looking for that. Here's one!
4: Use parcel ๐ฆ
parcel
is an alternative to webpack
that comes with a lot more built in, and a lot less to set up. As a matter of fact, you don't have to set up anything at all! Just install parcel
, run it, and ship your compiled code!
5: How about create-react-app? ๐ฟ
If you can't be bothered to set up and maintain your own build pipeline, you shouldn't. Facebook has created its own boilerplate generator, create-react-app
, that sets everything up for you.
You can read more about create-react-app
on its website, or in last year's article on it!
5: Use HTM! โ๏ธ
If the entire concept of transpiling your code is putting you off - or if you're just about that next level shit, code miracle worker Jason Miller has got your back. He's built a 700 byte (!) framework that basically implements JSX inside template strings. It looks like this:
import { html } from "htm";
const Heading = (props) => html` <h1>${props.children}</h1> `;
const App = () => html` <${Heading}>Hello world<//> `;
If you're about that build step life, you can even compile the entire library away! Now, that's pretty neat.
Have a merry JSXmas! ๐
So now you know a ton of ways to use JSX. If you want, you can even use JSX to do completely ridiculous things!
I hope you've learned something, and that you use the correct approach for your next encounter with this beautiful syntax extension. Thanks for reading!